Working with BAPIs
BAPIs (Business Application Programmers Interfaces) are the methods that get or modify the data of the ECC business objects. A sample object might be a purchase requisition and a sample method might be to create a new one.
This section contains several examples, both HEI and Scripted, of working with BAPIs.
BAPI_EMPLOYEE_GETLIST
This example gets the employees with last name starting with letter M.


BAPI_SALESORDER_GETLIST
Here is a typical select statement where the input values do not look the same as
they look if this BAPI were called from inside of the SAPGUI. For example, the
customer number is not 2300, as entered in the SAPGUI, it is 0000002300, as it
exists in table KNA1 in the database under ECC. The year format is defined as
YYYYMMDD from outside the SAPGUI, but regionally defined from within.


BAPI_VENDOR_FIND
This example shows an input table SELOPT_TAB being defined fin the Select
Statement.
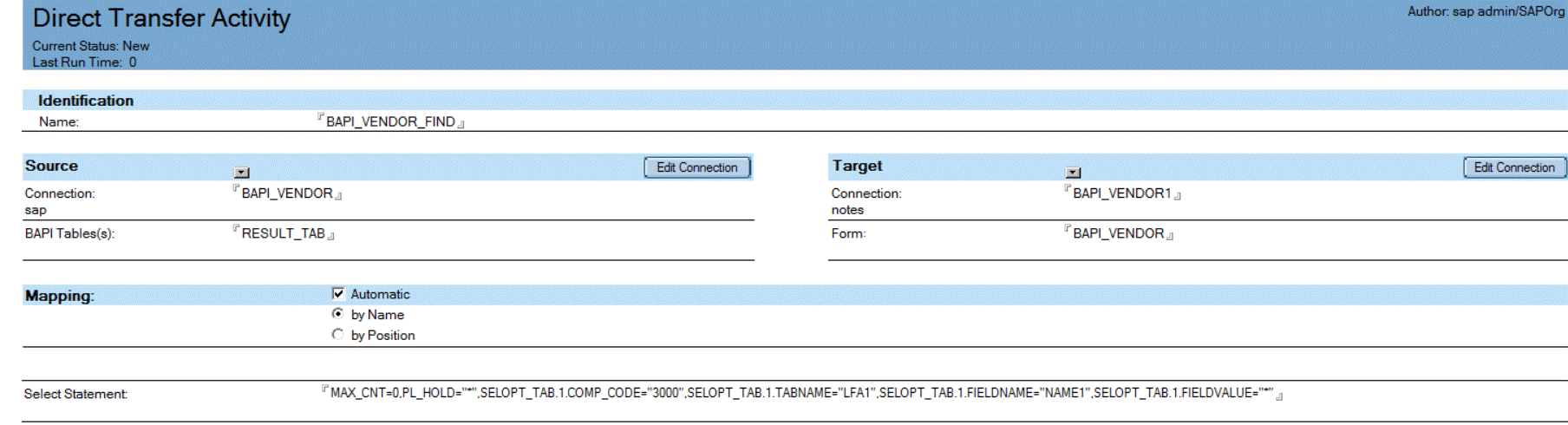
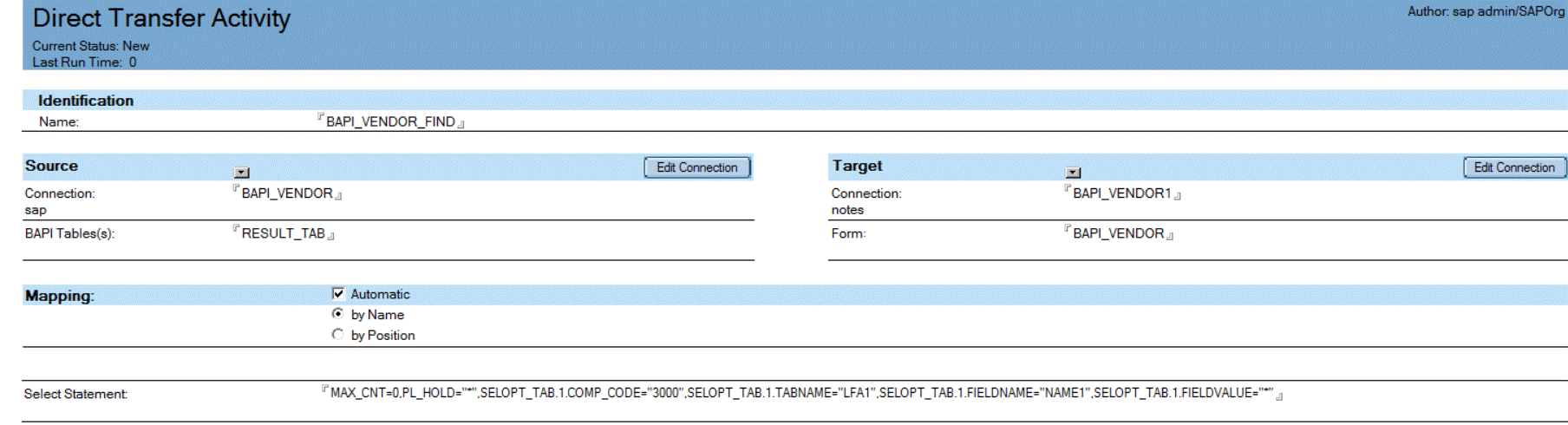
BAPI_REQUISITION_GETDETAIL
This example shows a DECS Virtual Fields activity calling a BAPI to get back
requisition item information.


Note: Using multi-value data fields allows you to get multiple rows from ECC and display
them in a single Notes document. That is why the fields on the Notes side above are
of type Binary, because they are lists (multi-value fields).

BAPI_REQUISITION_CREATE
In this example, a BAPI method is used with LC LSX. This example uses the LCConnecion
Call method. (Also see Example 6, below.) First an input fieldlist is set up and
then sent to ECC. After the call, the new Requisition Number is
returned.
Sub Initialize
Dim notessession As New NotesSession
Dim session As New LCSession
Dim target As New LCConnection("sap")
target.Database = "BAPI_REQUISITION_CREATE"
target.Userid = "muster"
target.Password = "ides"
target.Client="800"
target.Destination="LD3"
target.SystemNo=0
target.Language="EN"
target.Server = "sapaix.lotus.com"
target.Debuglevel = 0
target.Connect
On Error Goto errorhandler
Dim fldLst As New LCFieldList
Dim fldresult As New LCFieldList
Dim field As LCField
Dim number As New LCNumeric
target.Metadata = "*"
Set field = fldLst.Append ("REQUISITION_ACCOUNT_ASSIGNMENTCOST_CTR",LCTYPE_TEXT)
field.text = "0000001000" 'Look at the leading 0's,
' not visible in the SAPGUI but required.
Set field = fldLst.Append ("REQUISITION_ACCOUNT_ASSIGNMENTG_L_ACCT",LCTYPE_TEXT)
field.text = "0000400000" 'Look at the leading 0's,
' not visible in the SAPGUI but required.
number.text = "1"
Set field = fldLst.Append ("REQUISITION_ACCOUNT_ASSIGNMENTPREQ_ITEM",LCTYPE_NUMERIC)
Call field.setnumeric (1, number)
number.text = "7"
Set field = fldLst.Append ("REQUISITION_ACCOUNT_ASSIGNMENTPREQ_QTY",LCTYPE_NUMERIC)
Call field.setnumeric (1, number)
Set field = fldLst.Append ("REQUISITION_ITEMSACCTASSCAT", LCTYPE_TEXT)
field.value = "K"
Dim money As New LCCurrency
money.value = 75.00
Set field = fldLst.Append ("REQUISITION_ITEMSC_AMT_BAPI", LCTYPE_CURRENCY)
Call field.setcurrency (1, money)
Dim flddate As New LCDatetime (2001,05,04)
Set field = fldLst.Append ("REQUISITION_ITEMSDELIV_DATE", LCTYPE_DATETIME)
Call field.setdatetime(1, flddate)
Set field = fldLst.Append ("REQUISITION_ITEMSDOC_TYPE", LCTYPE_TEXT)
field.text = "NB"
Set field = fldLst.Append ("REQUISITION_ITEMSITEM_CAT", LCTYPE_TEXT)
field.text = "0"
Set field = fldLst.Append ("REQUISITION_ITEMSPLANT", LCTYPE_TEXT)
field.text = "1000"
number.text = "1"
Set field = fldLst.Append ("REQUISITION_ITEMSPREQ_ITEM", LCTYPE_NUMERIC)
Call field.setnumeric (1, number)
number.text = "7"
Set field = fldLst.Append ("REQUISITION_ITEMSQUANTITY", LCTYPE_NUMERIC)
Call field.setnumeric (1, number)
Set field = fldLst.Append ("REQUISITION_ITEMSSHORT_TEXT", LCTYPE_TEXT)
field.text = "MY REQ"
Set field = fldLst.Append ("REQUISITION_ITEMSCURRENCY", LCTYPE_TEXT)
field.text = "USD"
Set field = fldLst.Append ("REQUISITION_ITEMSUNIT", LCTYPE_TEXT)
field.text = "EA"
Set field = fldLst.Append ("REQUISITION_ITEMSMAT_GRP", LCTYPE_TEXT)
field.text = "00107"
Set field = fldLst.Append("REQUISITION_ITEMSPUR_GROUP", LCTYPE_TEXT)
field.text = "000"
Set field = fldLst.Append ("REQUISITION_ITEMSCREATED_BY", LCTYPE_TEXT)
field.text = "MUSTER"
Set field = fldLst.Append("REQUISITION_ITEMSPREQ_NAME",LCTYPE_TEXT)
field.text = "MUSTER"
Set field = fldLst.Append ("REQUISITION_ITEMSDISTRIB", LCTYPE_TEXT)
field.text = " "
Set field = fldLst.Append ("REQUISITION_ITEMSIR_IND", LCTYPE_TEXT)
field.text = "X"
Set field = fldLst.Append ("REQUISITION_ITEMSGR_IND", LCTYPE_TEXT)
field.text = "X"
Dim count, counter2 As Integer
session.ClearStatus
target.MapByName = True
count = target.call(fldLst, 1, fldresult)
Dim index As Long
call target.Fetch (fldresult, 1, 1)
Set field = fldresult.lookup("EXPORTSNUMBER", index)
Print "EXPORTSNUMBER = " & field.text (0)
Exit Sub
errorhandler:
Dim Msg As String
Dim Msgcode As Long
Dim status As Integer
Dim result As String
If session.status <> LCSUCCESS Then
status = session.GetStatus(result, Msgcode, Msg)
End If
Msgbox result
End Sub
BAPI_REQUISITION_CREATE
In this example, a BAPI method is used with the LC LSX. This example uses the
LCConnection Execute method. It has the advantage of allowing you to define more
than a single item in the Purchase Order. Look at the Select Statement for
details.
Sub Initialize
On Error Goto errorhandler
Dim notessession As New NotesSession
Dim session As New lcsession
Dim target As New LCConnection("sap")
target.Database = "BAPI_REQUISITION_CREATE"
target.Userid = "muster"
target.Password = "ides"
target.Client="800"
target.Destination="LD3"
target.SystemNo=0
target.Language="EN"
target.Server = "sapaix.lotus.com"
target.Debuglevel = 0
target.Connect
Dim fldLst As New LCFieldList
Dim fldresult As New LCFieldList
Dim field As LCField
Dim number As New LCNumeric
target.Metadata = "*"
Dim selectstatement As String
selectstatement = |REQUISITION_ACCOUNT_ASSIGNMENT.1.COST_CTR="0000001000",| +_
|REQUISITION_ACCOUNT_ASSIGNMENT.1.G_L_ACCT="0000400000",| +_
|REQUISITION_ACCOUNT_ASSIGNMENT.1.PREQ_ITEM=1,| +_
|REQUISITION_ACCOUNT_ASSIGNMENT.1.PREQ_QTY=7,| +_
|REQUISITION_ITEMS.1.ACCTASSCAT="K",| +_ 'First item
|REQUISITION_ITEMS.1.C_AMT_BAPI=75.00,| +_
|REQUISITION_ITEMS.1.DELIV_DATE="20010504",| +_
|REQUISITION_ITEMS.1.DOC_TYPE="NB",| +_
|REQUISITION_ITEMS.1.ITEM_CAT="0",| +_
|REQUISITION_ITEMS.1.PLANT="1000",| +_
|REQUISITION_ITEMS.1.PREQ_ITEM=1,| +_
|REQUISITION_ITEMS.1.QUANTITY=6,| +_
|REQUISITION_ITEMS.1.SHORT_TEXT="Item 1 Text",| +_
|REQUISITION_ITEMS.1.CURRENCY="USD",| +_
|REQUISITION_ITEMS.1.UNIT="EA",| +_
|REQUISITION_ITEMS.1.MAT_GRP="00107",| +_
|REQUISITION_ITEMS.1.PUR_GROUP="000",| +_
|REQUISITION_ITEMS.1.CREATED_BY="MUSTER",| +_
|REQUISITION_ITEMS.1.PREQ_NAME="MUSTER",| +_
|REQUISITION_ITEMS.1.DISTRIB=" ",| +_
|REQUISITION_ITEMS.1.IR_IND="X",| +_
|REQUISITION_ITEMS.1.GR_IND="X",| +_
|REQUISITION_ITEMS.2.ACCTASSCAT="K",| +_ 'Second item
|REQUISITION_ITEMS.2.C_AMT_BAPI=75.00,| +_
|REQUISITION_ITEMS.2.DELIV_DATE="20010504",| +_
|REQUISITION_ITEMS.2.DOC_TYPE="NB",| +_
|REQUISITION_ITEMS.2.ITEM_CAT="0",| +_
|REQUISITION_ITEMS.2.PLANT="1000",| +_
|REQUISITION_ITEMS.2.PREQ_ITEM=1,| +_
|REQUISITION_ITEMS.2.QUANTITY=7,| +_
|REQUISITION_ITEMS.2.SHORT_TEXT="Item 2 text",| +_
|REQUISITION_ITEMS.2.CURRENCY="USD",| +_
|REQUISITION_ITEMS.2.UNIT="EA",| +_
|REQUISITION_ITEMS.2.MAT_GRP="00107",| +_
|REQUISITION_ITEMS.2.PUR_GROUP="000",| +_
|REQUISITION_ITEMS.2.CREATED_BY="MUSTER",| +_
|REQUISITION_ITEMS.2.PREQ_NAME="MUSTER",| +_
|REQUISITION_ITEMS.2.DISTRIB=" ",| +_
|REQUISITION_ITEMS.2.IR_IND="X",| +_
|REQUISITION_ITEMS.2.GR_IND="X"|
Dim count, counter2 As Integer
session.ClearStatus
target.MapByName = True
count = target.execute(selectstatement, fldresult)
Dim index As Long
call target.Fetch (fldresult, 1, 1)
Set field = fldresult.lookup("EXPORTSNUMBER", index)
Print "EXPORTSNUMBER = " & field.text (0)
Exit Sub
errorhandler:
Dim Msg As String
Dim Msgcode As Long
Dim status As Integer
Dim result As String
If session.status <> LCSUCCESS Then
status = session.GetStatus(result, Msgcode, Msg)
End If
Msgbox result
End Sub