Custom Widget API
This API provides a mechanism to incorporate custom widgets into the HCL Domino Leap product.
Getting started
Product Configuration
Additional resources can be loaded into the Domino Leap UI's by adding runtimeResources settings to Domino Leap Configuration Settings NSF file (VoltConfig.nsf). For more information, see Domino Leap configuration settings.
These additional resources are expected to include definitions of your custom widgets and any auxiliary styles or libraries that are required to support them.
For example: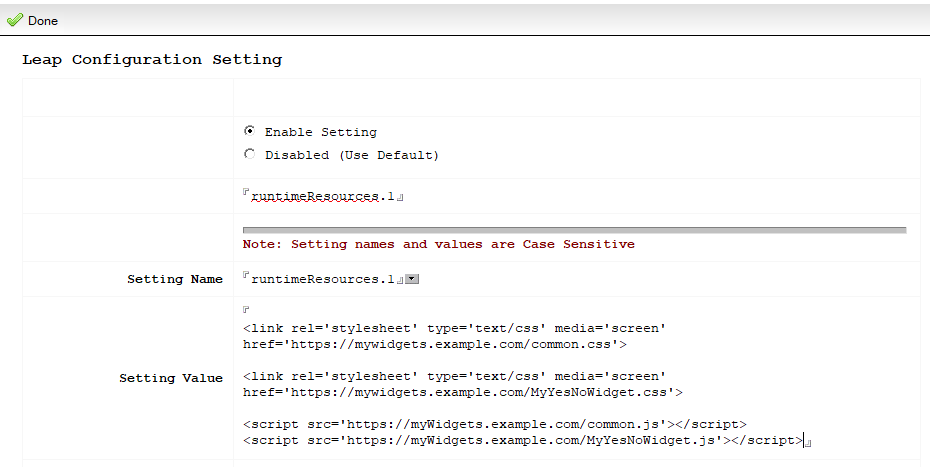
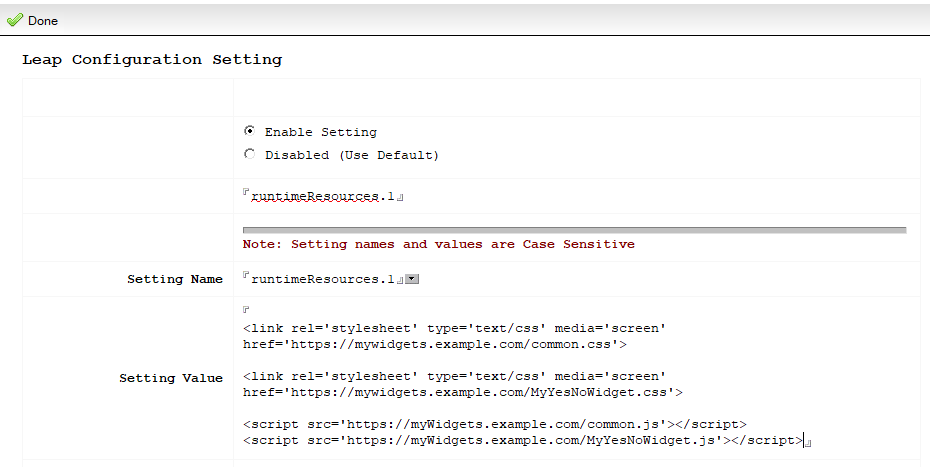
Registering a Widget
As your custom .js is loaded into the page, it is expected to register one or more
widget
definitions:
const myWidgetDefinition = {...};
nitro.registerWidget(myWidgetDefintion);
Full descriptions and examples are provided in the following topics. The following
example is the basic skeleton of a custom
widget:
const myWidgetDefinition = {
id: 'example.YesNo', // uniquely identifies this widget
version: '2.0.0', // the widget's version
apiVersion: '1.0.0', // the version of this API
label: 'Yes/No',
description: 'Allows user to choose "Yes" or "No"',
datatype: {
type: 'string' // must be one of 'string', 'date', 'number', 'boolean', time, timestamp
},
// for placement in the palette
category: {
id: 'example.choice.widgets',
label: 'Choice Components'
},
iconClassName: 'myYesNoIcon', // styling of this class expected in custom .css
builtInProperties: [...], // use existing properties: 'title', 'required', etc
properties: [...], // custom properties, of prescribed types
// called by Leap to initialize widget in the DOM with initial properties, and set-up event handling
instantiate: function (context, domNode, initialProps, eventManager) {
return {
// (optional) for display in various parts of the UI
getDisplayTitle: function () {
return ...
},
// (required) for Leap to get widget's data value
getValue: function () {
return ...
},
// (required) for Leap to set widget's data value
setValue: function (val) {
...
},
// (optional) for additional validation of value
validateValue: function (val) {
// return true, false, or custom error message
},
// (required) called when properties change in the authoring environment, or via JavaScript API
setProperty: function (propName, propValue) {
...
},
// (optional) method to enable/disable widget
setDisabled: function (isDisabled) {
...
},
// (optional) determines what the author can do with the widget via custom JavaScript
getJSAPIFacade: function () {
return {
...
};
}
};
}
};