Example of OpenWhisk Action
As a detailed example, suppose you want to create an OpenWhisk action to integrate with an API provided by the company, Skyscanner, to find out various flight options between a Source Place and a Destination Place on a particular date.
Also consider you want provides various other input parameters like cabin class, number of passengers etc.
You can use the OpenWhisk module to create the function as shown in the screenshot below. Also, the full source code of the function is given right below:
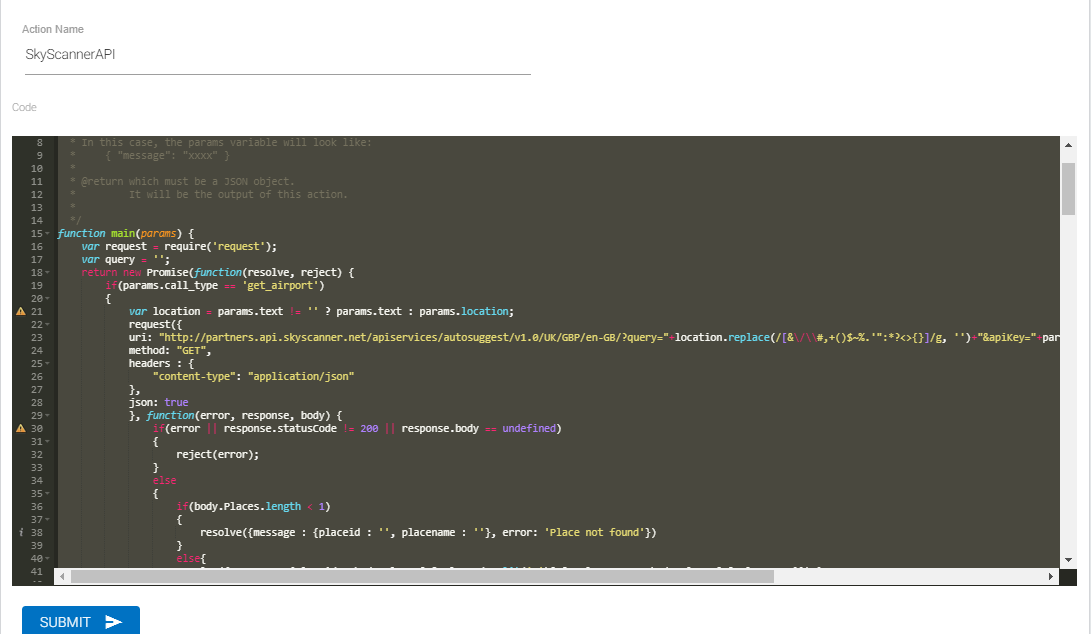
/**
*
* main () will be invoked when you Run This Action.
*
* @param Whisk actions accept a single parameterparameter,
* which must be a JSON object.
*
* In this case, the params variable will look like:
* { "message": "xxxx" }
*
* @return which must be a JSON object.
* It will be the output of this action.
*
*/
function main(params) {
var request = require('request');
var query = '';
return new Promise (function(resolve, reject) {
if(params.call_type == 'get_airport')
{
var location = params.text != '' ? params.text : params.location;
request({
uri: "http://partners.api.skyscanner.net/apiservices/autosuggest/v1.0/UK/GBP/en-GB/?query="+location.replace(/[&\/\\#,+()$~%.'":*?<>{}]/g, '')+"&apiKey="+params.apikey,
method: "GET",
headers: {
"content-type": "application/json"
},
json: true
}, function(error, response, body) {
if(error || response.statusCode != 200 || response.body == undefined)
{
reject(error);
}
else
{
if(body.Places.length < 1)
{
resolve({message : {placeid : '', placename : ''}, error: 'Place not found'})
}
else{
resolve({message : {placeid : body.Places[0].PlaceId.split('-')[0], placename : body.Places[0].PlaceName}});}
}
});
}
else if(params.call_type == 'flight_details')
{
var date_new;
if(params.date)
{
console.log(params.date);
date_new = params.date;
}
else
{
var date_array = params.text.split('-');
date_new = date_array[2]+'-'+date_array[1]+'-'+date_array[0]
}
params.outbounddate = date_new;
console.log('Calling create session');
request({
uri: 'http://partners.api.skyscanner.net/apiservices/pricing/v1.0',
method: "POST",
headers : {
"content-type": "application/x-www-form-urlencoded"
},
form: {
"cabinclass": params.cabinclass,
"country": params.country,
"currency": params.currency,
"locale": params.locale,
"locationSchema": params.locationSchema,
"originplace": params.originplace,
"destinationplace": params.destinationplace,
"outbounddate": params.outbounddate,
"adults": params.adults,
"children": params.children,
"infants": params.infants,
"apikey": params.apikey
},
json: true
}, function(error, response, body) {
console.log(response.statusCode);
console.log(body);
if(error || response.statusCode != 201)
{
reject(error)
}
else
{
var session_location = response.headers.location.split('/');
var session = session_location[session_location.length-1]
console.log(session);
//resolve({message : session, url : response.headers});
request({
uri: "http://partners.api.skyscanner.net/apiservices/pricing/sg1/v1.0/"+session+"?apikey="+params.apikey+"&sortType=price&sortOrder=asc",
method: "GET",
headers : {
"content-type": "application/json"
},
json: true
}, function(error, response, body) {
console.log(error);
console.log(response.statusCode);
if(error || response.statusCode != 200 || response.body == undefined)
{
reject(error);
}
else
{
var top_three_itineraries = body.Itineraries.slice(0,3);
var agents = body.Agents;
var carriers = body.Carriers;
var segments = body.Segments;
var legs = body.Legs;
var flights = [];
var content = '<style>my-result-list .table-listing.my-res-table { display: block;width: 100%;float: left;}'+
'.table-listing {display: table;width: 100%;table-layout: fixed;border-collapse: separate;}' +
'ul li{display: inline;padding:15px;}' +
'li {display: table;min-height : 45px;font-weight: bold;}' +
'li div {height:45px;display:table-cell;vertical-align : middle;text-align: center;}' +
'.inner img{height: 40px;}' +
'.flight-div{background: #e0f7fa;padding:1px 0 1px 0;border-radius: 12px;}'+
'</style>';
for(var itinerary in top_three_itineraries)
{
content += '<div class="flight-div"><ul class="table-listing my-res-table">';
var moment = require('moment');
flights[itinerary] = {};
flights[itinerary].pricingoptions = top_three_itineraries[itinerary]['PricingOptions'][0];
flights[itinerary].agent = getObjectFromArray('Id',flights[itinerary].pricingoptions, agents);
flights[itinerary].leg = getObjectFromArray('Id',top_three_itineraries[itinerary].OutboundLegId,legs)
flights[itinerary].carrier = getObjectFromArray('Id',flights[itinerary].leg.Carriers[0], carriers);
flights[itinerary].segment = getObjectFromArray('Id',flights[itinerary].leg.SegmentIds[0], segments);
content += '<li><div class="outer"><div class="middle"><div class="inner">';
content += '<img src="'+flights[itinerary].carrier.ImageUrl+'" />';
content += '</div></div></div></li>';
content += '<li><div class="outer"><div class="middle"><div class="inner">';
content += '<small>Departure</small> <br/>'+moment(flights[itinerary].segment.DepartureDateTime).format("dddd, MMMM Do YYYY, h:mm a");
content += '</div></div></div></li>';
content += '<li><div class="outer"><div class="middle"><div class="inner">';
content += '<small>Arrival</small> <br/>'+moment(flights[itinerary].segment.ArrivalDateTime).format("dddd, MMMM Do YYYY, h:mm a");
content += '</div></div></div></li>';
content += '<li><div class="outer"><div class="middle"><div class="inner">';
content += ' $ '+flights[itinerary].pricingoptions.Price;
content += '</div></div></div></li>';
var duration = flights[itinerary].segment.Duration;
var hours = Math.floor( duration / 60);
var minutes = duration % 60;
content += '<li><div class="outer"><div class="middle"><div class="inner">';
content += hours+'h '+minutes+'m';
content += '</div></div></div></li>';
content += '<li><div class="outer"><div class="middle"><div class="inner">';
content += '<a href="'+flights[itinerary].pricingoptions.DeeplinkUrl+'" target="_blank">Book Now</a>';
content += '</div></div></div></li>';
content += '</ul></div>';
}
resolve({message : content, flight_hash: flights});
}
});
}
});
}
else if(params.call_type == 'flight_cache')
{
var date_new;
if(params.date)
{
console.log(params.date);
date_new = params.date;
}
else
{
var date_array = params.text.split('-');
date_new = date_array[2]+'-'+date_array[1]+'-'+date_array[0]
}
console.log("http://partners.api.skyscanner.net/apiservices/browsequotes/v1.0/IN/USD/en-US/"+params.originplace+"/"+params.destinationplace+"/"+date_new+"?apikey="+params.apikey+"&sortType=price&sortOrder=asc");
request({
uri: "http://partners.api.skyscanner.net/apiservices/browsequotes/v1.0/IN/USD/en-US/"+params.originplace+"/"+params.destinationplace+"/"+date_new+"?apikey="+params.apikey+"&sortType=price&sortOrder=asc",
method: "GET",
headers : {
"content-type": "application/json"
},
json: true
}, function(error, response, body) {
if(error || response.statusCode != 200 || response.body == undefined)
{
resolve({message : 'Sorry, I could not find a flight for you...<br/><br/>'});
}
else
{
var quote = body.Quotes[0];
var price = quote.MinPrice;
var carrier_obj = getObjectFromArray('CarrierId',quote.OutboundLeg.CarrierIds[0],body.Carriers);
var carrier = carrier_obj.Name;
var origin = getObjectFromArray('PlaceId',quote.OutboundLeg.OriginId,body.Places);
var origin_name = origin.Name + '('+origin.IataCode+')';
var destination = getObjectFromArray('PlaceId',quote.OutboundLeg.DestinationId,body.Places);
var destination_name = destination.Name + '('+destination.IataCode+')';
var direct = quote.Direct ? '<b/>direct</b>' : '';
var price_actual = body.Currencies[0].Symbol + price;
if(body.Quotes.length > 1)
{
var content = '<table style="width:100%; border-collapse:collapse"><tbody><tr style="background-color: #eee"><th>Airlines</th><th>Direct Flight</th><th>Origin</th><th>Destination</th><th>Price</th></tr>'
for(var quote in body.Quotes)
{
content += '<tr>';
content += '<td style="border: 1px solid black;">'+getObjectFromArray('CarrierId',body.Quotes[quote].OutboundLeg.CarrierIds[0],body.Carriers).Name+'</td>';
content += '<td style="border: 1px solid black;">'+(body.Quotes[quote].Direct ? "Yes" : "No")+'</td>';
content += '<td style="border: 1px solid black;">'+getObjectFromArray('PlaceId',body.Quotes[quote].OutboundLeg.OriginId,body.Places).Name+'</td>';
content += '<td style="border: 1px solid black;">'+getObjectFromArray('PlaceId',body.Quotes[quote].OutboundLeg.DestinationId,body.Places).Name+'</td>';
content += '<td style="border: 1px solid black;"> $'+body.Quotes[quote].MinPrice+'</td>';
content += '</tr>';
}
content += '</tbody></table>';
resolve({message: 'Great, There are some flight from <b>'+origin_name+'</b> to <b>'+destination_name+'</b> Please find those options below.<br/><br/>'+content+'<br/><br/>'});
}
else if(body.Quotes.length == 1)
{
resolve({message: 'Great, There is a '+direct+' flight from <b>'+origin_name+'</b> to <b>'+destination_name+'</b> at a minimum price of <b>'+price_actual+'</b> by <b>'+carrier+'</b> airlines.<br/><br/>'});
}
else
{
resolve({message : 'Sorry, I could not find a flight for you.<br/><br/>'});
}
//resolve({message : body});
}
});
}
});
}
function getObjectFromArray(key, value, array)
{
for(element in array)
{
if(array[element][key] == value)
{
return array[element]
}
}
return false;
}